フックを設定する
src/index.js
import "./logged-in";
src/logged-in.js
import { addFilter } from "@wordpress/hooks";
import { createHigherOrderComponent } from "@wordpress/compose";
import { InspectorControls } from "@wordpress/block-editor";
export const withInspectorControls = createHigherOrderComponent(
// BlockEditは元のブロックのコンポーネント
(BlockEdit) => (props) => {
return (
<>
<BlockEdit {...props} />
{/* ブロックの構成に対し自由テキストなどを追加する */}
ブロックの直後に追加するテキスト
<InspectorControls>右設定パネルに追加するテキスト</InspectorControls>
</>
);
},
);
// 第一引数
// editor.BlockEditフック:ブロック エディターで編集中にブロックの動作を変更を掛ける。すべてのブロックに対して実行される
// 第二引数:一意の名称
// 第三引数:関数
addFilter(
"editor.BlockEdit",
"gtt/editor/logged-in/with-inspector-controls",
withInspectorControls,
);
エディター
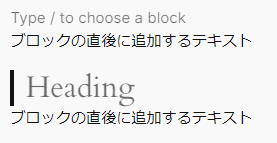
設定パネル
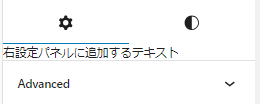
設定パネルにトグルを作成する
src/logged-in.js
import { addFilter } from "@wordpress/hooks";
import { PanelBody, ToggleControl } from "@wordpress/components";
import { createHigherOrderComponent } from "@wordpress/compose";
import { InspectorControls } from "@wordpress/block-editor";
export const withInspectorControls = createHigherOrderComponent(
(BlockEdit) => (props) => {
// attributesはpropsの中身
const {
attributes: { gttIsLogin },
setAttributes,
} = props;
return (
<>
<BlockEdit {...props} />
ブロックの直後に追加するテキスト
<InspectorControls>
<PanelBody title="ログインユーザー非表示設定">
<ToggleControl
label="ログインユーザー"
// チェック状態を識別するデータの名称をgttIsLoginとする
checked={gttIsLogin}
onChange={(checked) => setAttributes({ gttIsLogin: checked })}
/>
</PanelBody>
</InspectorControls>
</>
);
},
);
addFilter(
"editor.BlockEdit",
"gtt/editor/logged-in/with-inspector-controls",
withInspectorControls,
);
attributesを登録する
src/logged-in.js
// settingsは各ブロックに設定可能なスタイル情報
export function addAttribute(settings) {
settings.attributes = {
...settings.attributes,
// attributesにgttIsLoginを追加
gttIsLogin: {
type: "boolean",
},
};
return settings;
}
...
addFilter(
"blocks.registerBlockType",
"gtt/logged-in/add-attributes",
addAttribute,
);
動的処理を記述する
block-customize.php
require plugin_dir_path(__FILE__). 'includes/logged-in.php';
includes/logged-in.php
<?php
function gtt_add_attributes_is_logged_in($settings){
$attributes = array();
if(!empty($settings['attributes'])){
$attributes = $settings['attributes'];
}
$add_attributes = array(
'gttIsLogin'=> array(
'type' => 'boolean',
'default'=> false
),
);
$settings['attributes'] = array_merge(
$attributes,
$add_attributes
);
return $settings;
}
add_filter('block_type_metadata_settings','gtt_add_attributes_is_logged_in');
function gtt_render_block_logged_in($block_content, $block){
if( ! empty($block['attrs']['gttIsLogin']) && ! is_user_logged_in()){
return null;
}
return $block_content;
};
add_filter('render_block', 'gtt_render_block_logged_in',10, 2);